Java Read a file from resources folder
By:Roy.LiuLast updated:2019-08-17
In this tutorial, we will show you how to read a file from a resources folder or classpath, in both runtime and unit test environment. Try putting a file into the src/main/resources folder, and read the file with following code snippets :
1. getResource
File file = new File( getClass().getClassLoader().getResource("database.properties").getFile() );
2. getResourceAsStream
InputStream inputStream = getClass() .getClassLoader().getResourceAsStream("database.properties");
Note
You may interest at this getResourceAsStream in static method
You may interest at this getResourceAsStream in static method
1. Project Directory
Review a Maven project Structure.
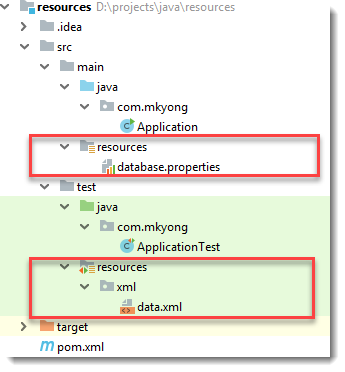
2. Read file from resources folder
src/main/resources/database.properties
datasource.url=jdbc:mysql://localhost/mkyong?useSSL=false datasource.username=root datasource.password=password datasource.driver-class-name=com.mysql.jdbc.Driver
Read database.properties file and print out the content.
Application.java
package com.mkyong; import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.net.URL; public class Application { public static void main(String[] args) throws IOException { Application main = new Application(); File file = main.getFileFromResources("database.properties"); printFile(file); // get file from classpath, resources folder private File getFileFromResources(String fileName) { ClassLoader classLoader = getClass().getClassLoader(); URL resource = classLoader.getResource(fileName); if (resource == null) { throw new IllegalArgumentException("file is not found!"); } else { return new File(resource.getFile()); private static void printFile(File file) throws IOException { if (file == null) return; try (FileReader reader = new FileReader(file); BufferedReader br = new BufferedReader(reader)) { String line; while ((line = br.readLine()) != null) { System.out.println(line);
Output
datasource.url=jdbc:mysql://localhost/mkyong?useSSL=false datasource.username=root datasource.password=password datasource.driver-class-name=com.mysql.jdbc.Driver
3. Unit Test
src/test/resources/xml/data.xml
<test> <case id='1'> <param>100</param> <expected>mkyong</expected> </case> <case id='2'> <param>99</param> <expected>mkyong</expected> </case> </test>
Read data.xml and print out the content.
ApplicationTest.java
package com.mkyong; import org.apache.commons.io.IOUtils; import org.junit.jupiter.api.DisplayName; import org.junit.jupiter.api.Test; import java.io.IOException; import java.io.InputStream; import java.nio.charset.StandardCharsets; public class ApplicationTest { @DisplayName("Test loading XML") @Test void loadXMLTest() { ClassLoader classLoader = getClass().getClassLoader(); try (InputStream inputStream = classLoader.getResourceAsStream("xml/data.xml")) { String result = IOUtils.toString(inputStream, StandardCharsets.UTF_8); System.out.println(result); } catch (IOException e) { e.printStackTrace();
Output
<test> <case id='1'> <param>100</param> <expected>mkyong</expected> </case> <case id='2'> <param>99</param> <expected>mkyong</expected> </case> </test>
pom.xml
<dependencies> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.6</version> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter</artifactId> <version>5.4.2</version> <scope>test</scope> </dependency> </dependencies>
From:一号门
Previous:jsoup : Send search query to Google
COMMENTS