Java RMI Hello World example
RMI stands for Remote Method Invocation and it is the object-oriented equivalent of RPC (Remote Procedure Calls). RMI was designed to make the interaction between applications using the object-oriented model and run on different machines seem like that of stand-alone programs.
The code below will give you the basis to Java RMI with a very simple example of a Server-Client communication model.
1. The Remote Interface
The first thing we have to design is the Remote Interface that both Server and Client will implement. The Interface must always be public and extend Remote. All methods described in the Remote interface must list RemoteException in their throws clause.
Our RMIInterface has only one method; it receives a String parameter and returns String.
package com.mkyong.rmiinterface; import java.rmi.Remote; import java.rmi.RemoteException; public interface RMIInterface extends Remote { public String helloTo(String name) throws RemoteException;
2. The Server
Our Server extends UnicastRemoteObject and implements the Interface we made before. In the main method we bind the server on localhost with the name “MyServer”.
package com.mkyong.rmiserver; import java.rmi.Naming; import java.rmi.RemoteException; import java.rmi.server.UnicastRemoteObject; import com.mkyong.rmiinterface.RMIInterface; public class ServerOperation extends UnicastRemoteObject implements RMIInterface{ private static final long serialVersionUID = 1L; protected ServerOperation() throws RemoteException { super(); @Override public String helloTo(String name) throws RemoteException{ System.err.println(name + " is trying to contact!"); return "Server says hello to " + name; public static void main(String[] args){ try { Naming.rebind("//localhost/MyServer", new ServerOperation()); System.err.println("Server ready"); } catch (Exception e) { System.err.println("Server exception: " + e.toString()); e.printStackTrace();
3. The Client
Finally, we create the Client. To “find” the Server, the Client uses an RMIInterface Object that “looks” for a reference for the remote object associated with the name we pass as parameter. With that RMIInterface object we can now talk to the Server and receive responses.
package com.mkyong.rmiclient; import java.net.MalformedURLException; import java.rmi.Naming; import java.rmi.NotBoundException; import java.rmi.RemoteException; import javax.swing.JOptionPane; import com.mkyong.rmiinterface.RMIInterface; public class ClientOperation { private static RMIInterface look_up; public static void main(String[] args) throws MalformedURLException, RemoteException, NotBoundException { look_up = (RMIInterface) Naming.lookup("//localhost/MyServer"); String txt = JOptionPane.showInputDialog("What is your name?"); String response = look_up.helloTo(txt); JOptionPane.showMessageDialog(null, response);
4. How to run it
4.1 Create the three java files with your favorite IDE or Download the code below. Navigate to your source folder as shown below.
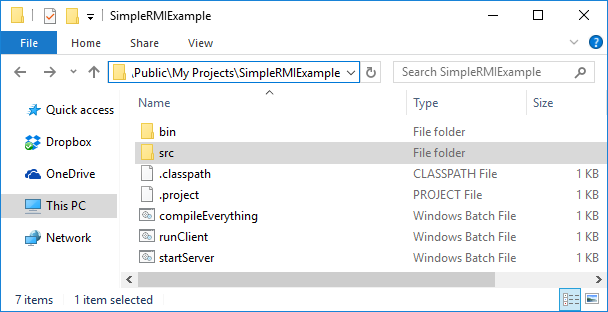
4.2 First thing we need to do is compile our sources. Run compileEverything.bat if you downloaded the code below or open a command window at your directory and run:
javac src/com/mkyong/rmiinterface/RMIInterface.java src/com/mkyong/rmiserver/ServerOperation.java src/com/mkyong/rmiclient/ClientOperation.java
4.3 Confirm that your sources were compiled by accessing their respective directories:
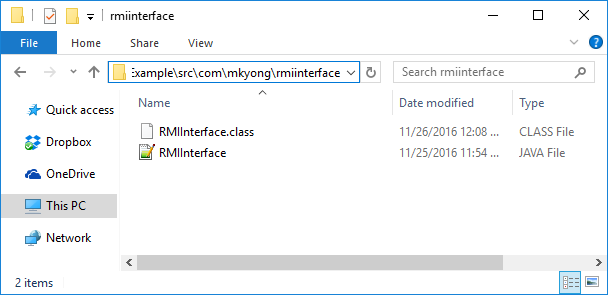
4.4 Next we need to start the rmiregistry. Again either run the startServer.bat or open a command window and run:
cd src start rmiregistry java com.mkyong.rmiserver.ServerOperation
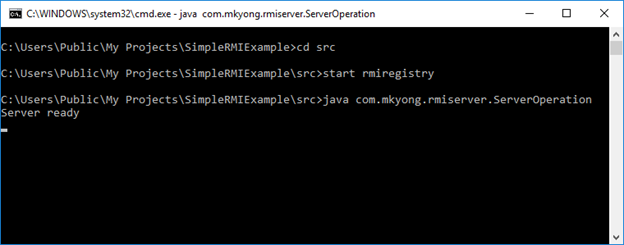
4.5 If RmiRegistry started successfully, there will be another window that looks like this:
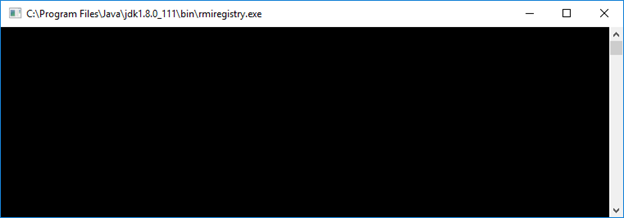
4.6 Now we are ready to run our Client:
Open a new command prompt window (or run the runClient.bat from the downloaded files) and run this:
cd src java com.mkyong.rmiclient.ClientOperation
The ClientOperation runs and prompts us for input:
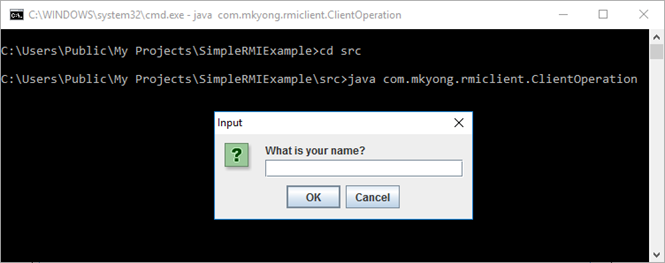
We type a name in the input field (ex. “Marilena”) and click “OK”
4.7 On Server side we can see this:
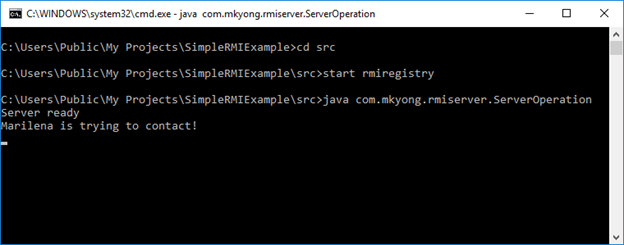
4.8 And on the Client side this:
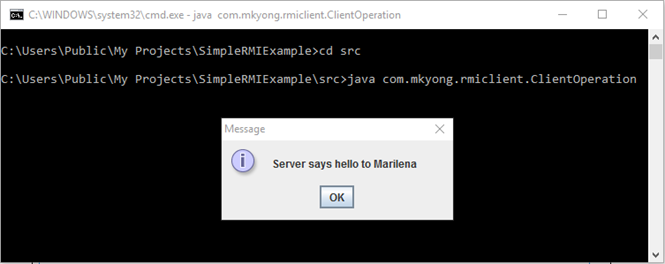
4.9 The client closes after the exchange is over, while the server stays on and we can communicate again if we run the client file again. Indeed, we run the client again and:
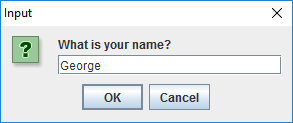
Server :
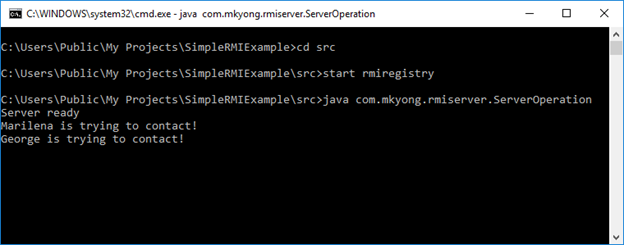
Client :
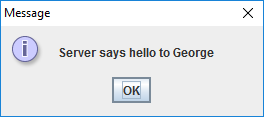
References
From:一号门
COMMENTS