Spring Boot Hello World Example Thymeleaf
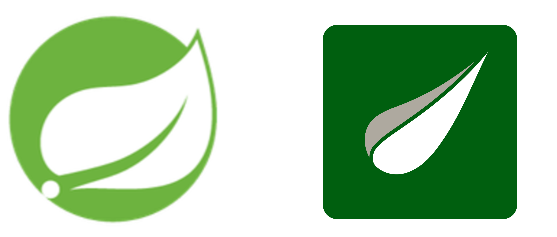
In this article, we will show you how to develop a Spring Boot web application, using Thymeleaf view, embedded Tomcat and package it as an executable JAR file.
Technologies used :
- Spring Boot 2.1.2.RELEASE
- Spring 5.1.4.RELEASE
- Thymeleaf 3.0.11.RELEASE
- Tomcat embed 9.0.14
- JUnit 4.12
- Maven 3
- Java 8
1. Project Directory
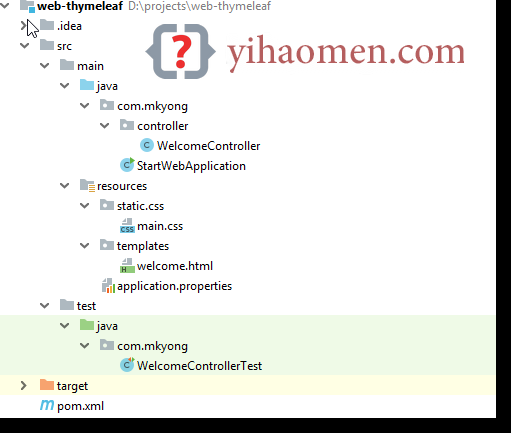
2. Maven
Put spring-boot-starter-web and spring-boot-starter-thymeleaf, it will get anything we need to develop a Spring MVC + Thymeleaf web application, including the embedded Tomcat server.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <artifactId>web-thymeleaf</artifactId> <packaging>jar</packaging> <name>Spring Boot Web Thymeleaf Example</name> <description>Spring Boot Web Thymeleaf Example</description> <version>1.0</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.2.RELEASE</version> </parent> <properties> <java.version>1.8</java.version> <bootstrap.version>4.2.1</bootstrap.version> </properties> <dependencies> <!-- web mvc --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- thymeleaf --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <!-- test --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!-- hot swapping, disable cache for template, enable live reload --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency> <!-- Optional, for bootstrap --> <dependency> <groupId>org.webjars</groupId> <artifactId>bootstrap</artifactId> <version>${bootstrap.version}</version> </dependency> </dependencies> <build> <plugins> <!-- Package as an executable jar/war --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <configuration> <addResources>true</addResources> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.0</version> </plugin> </plugins> </build> </project>
Display the project dependencies.
$ mvn dependency:tree [INFO] org.springframework.boot:web-thymeleaf:jar:1.0 [INFO] +- org.springframework.boot:spring-boot-starter-web:jar:2.1.2.RELEASE:compile [INFO] | +- org.springframework.boot:spring-boot-starter:jar:2.1.2.RELEASE:compile [INFO] | | +- org.springframework.boot:spring-boot-starter-logging:jar:2.1.2.RELEASE:compile [INFO] | | | +- ch.qos.logback:logback-classic:jar:1.2.3:compile [INFO] | | | | \- ch.qos.logback:logback-core:jar:1.2.3:compile [INFO] | | | +- org.apache.logging.log4j:log4j-to-slf4j:jar:2.11.1:compile [INFO] | | | | \- org.apache.logging.log4j:log4j-api:jar:2.11.1:compile [INFO] | | | \- org.slf4j:jul-to-slf4j:jar:1.7.25:compile [INFO] | | +- javax.annotation:javax.annotation-api:jar:1.3.2:compile [INFO] | | \- org.yaml:snakeyaml:jar:1.23:runtime [INFO] | +- org.springframework.boot:spring-boot-starter-json:jar:2.1.2.RELEASE:compile [INFO] | | +- com.fasterxml.jackson.core:jackson-databind:jar:2.9.8:compile [INFO] | | | +- com.fasterxml.jackson.core:jackson-annotations:jar:2.9.0:compile [INFO] | | | \- com.fasterxml.jackson.core:jackson-core:jar:2.9.8:compile [INFO] | | +- com.fasterxml.jackson.datatype:jackson-datatype-jdk8:jar:2.9.8:compile [INFO] | | +- com.fasterxml.jackson.datatype:jackson-datatype-jsr310:jar:2.9.8:compile [INFO] | | \- com.fasterxml.jackson.module:jackson-module-parameter-names:jar:2.9.8:compile [INFO] | +- org.springframework.boot:spring-boot-starter-tomcat:jar:2.1.2.RELEASE:compile [INFO] | | +- org.apache.tomcat.embed:tomcat-embed-core:jar:9.0.14:compile [INFO] | | +- org.apache.tomcat.embed:tomcat-embed-el:jar:9.0.14:compile [INFO] | | \- org.apache.tomcat.embed:tomcat-embed-websocket:jar:9.0.14:compile [INFO] | +- org.hibernate.validator:hibernate-validator:jar:6.0.14.Final:compile [INFO] | | +- javax.validation:validation-api:jar:2.0.1.Final:compile [INFO] | | +- org.jboss.logging:jboss-logging:jar:3.3.2.Final:compile [INFO] | | \- com.fasterxml:classmate:jar:1.4.0:compile [INFO] | +- org.springframework:spring-web:jar:5.1.4.RELEASE:compile [INFO] | | \- org.springframework:spring-beans:jar:5.1.4.RELEASE:compile [INFO] | \- org.springframework:spring-webmvc:jar:5.1.4.RELEASE:compile [INFO] | +- org.springframework:spring-aop:jar:5.1.4.RELEASE:compile [INFO] | +- org.springframework:spring-context:jar:5.1.4.RELEASE:compile [INFO] | \- org.springframework:spring-expression:jar:5.1.4.RELEASE:compile [INFO] +- org.springframework.boot:spring-boot-starter-thymeleaf:jar:2.1.2.RELEASE:compile [INFO] | +- org.thymeleaf:thymeleaf-spring5:jar:3.0.11.RELEASE:compile [INFO] | | +- org.thymeleaf:thymeleaf:jar:3.0.11.RELEASE:compile [INFO] | | | +- org.attoparser:attoparser:jar:2.0.5.RELEASE:compile [INFO] | | | \- org.unbescape:unbescape:jar:1.1.6.RELEASE:compile [INFO] | | \- org.slf4j:slf4j-api:jar:1.7.25:compile [INFO] | \- org.thymeleaf.extras:thymeleaf-extras-java8time:jar:3.0.2.RELEASE:compile [INFO] +- org.springframework.boot:spring-boot-starter-test:jar:2.1.2.RELEASE:test [INFO] | +- org.springframework.boot:spring-boot-test:jar:2.1.2.RELEASE:test [INFO] | +- org.springframework.boot:spring-boot-test-autoconfigure:jar:2.1.2.RELEASE:test [INFO] | +- com.jayway.jsonpath:json-path:jar:2.4.0:test [INFO] | | \- net.minidev:json-smart:jar:2.3:test [INFO] | | \- net.minidev:accessors-smart:jar:1.2:test [INFO] | | \- org.ow2.asm:asm:jar:5.0.4:test [INFO] | +- junit:junit:jar:4.12:test [INFO] | +- org.assertj:assertj-core:jar:3.11.1:test [INFO] | +- org.mockito:mockito-core:jar:2.23.4:test [INFO] | | +- net.bytebuddy:byte-buddy:jar:1.9.7:test [INFO] | | +- net.bytebuddy:byte-buddy-agent:jar:1.9.7:test [INFO] | | \- org.objenesis:objenesis:jar:2.6:test [INFO] | +- org.hamcrest:hamcrest-core:jar:1.3:test [INFO] | +- org.hamcrest:hamcrest-library:jar:1.3:test [INFO] | +- org.skyscreamer:jsonassert:jar:1.5.0:test [INFO] | | \- com.vaadin.external.google:android-json:jar:0.0.20131108.vaadin1:test [INFO] | +- org.springframework:spring-core:jar:5.1.4.RELEASE:compile [INFO] | | \- org.springframework:spring-jcl:jar:5.1.4.RELEASE:compile [INFO] | +- org.springframework:spring-test:jar:5.1.4.RELEASE:test [INFO] | \- org.xmlunit:xmlunit-core:jar:2.6.2:test [INFO] | \- javax.xml.bind:jaxb-api:jar:2.3.1:test [INFO] | \- javax.activation:javax.activation-api:jar:1.2.0:test [INFO] +- org.springframework.boot:spring-boot-devtools:jar:2.1.2.RELEASE:compile (optional) [INFO] | +- org.springframework.boot:spring-boot:jar:2.1.2.RELEASE:compile [INFO] | \- org.springframework.boot:spring-boot-autoconfigure:jar:2.1.2.RELEASE:compile [INFO] \- org.webjars:bootstrap:jar:4.2.1:compile [INFO] +- org.webjars:jquery:jar:3.0.0:compile [INFO] \- org.webjars:popper.js:jar:1.14.3:compile
3. Developer Tools
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency>
This spring-boot-devtools helps to disable the caches and enable hot swapping so that developers will always see the last changes. Good for development. Read this – Spring Boot – Developer tools Try to modify the Thymeleaf templates or properties files, refresh the browser to see the changes take effect immediately.
- For Eclipse IDE, it is integrated well with spring-boot-devtools.
- For Intellij IDEA, extra steps are required, read this reload static file is not working
4. Spring Boot + MVC
4.1 A simple controller.
package com.mkyong.controller; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam; import java.util.Arrays; import java.util.List; @Controller public class WelcomeController { // inject via application.properties @Value("${welcome.message}") private String message; private List<String> tasks = Arrays.asList("a", "b", "c", "d", "e", "f", "g"); @GetMapping("/") public String main(Model model) { model.addAttribute("message", message); model.addAttribute("tasks", tasks); return "welcome"; //view // /hello?name=kotlin @GetMapping("/hello") public String mainWithParam( @RequestParam(name = "name", required = false, defaultValue = "") String name, Model model) { model.addAttribute("message", name); return "welcome"; //view
4.2 Create a class and annotate with @SpringBootApplication. In IDE, run this class to start the entire web application.
package com.mkyong; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class StartWebApplication { public static void main(String[] args) { SpringApplication.run(StartWebApplication.class, args);
5. Thymeleaf + Static files
Read this Spring Boot Serving static content to understand the resource mapping.
5.1 For Thymeleaf template files, put in src/main/resources/templates/
<!DOCTYPE HTML> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Spring Boot Thymeleaf Hello World Example</title> <link rel="stylesheet" th:href="@{webjars/bootstrap/4.2.1/css/bootstrap.min.css}"/> <link rel="stylesheet" th:href="@{/css/main.css}"/> </head> <body> <nav class="navbar navbar-expand-md navbar-dark bg-dark fixed-top"> <a class="navbar-brand" href="#">Mkyong.com</a> </nav> <main role="main" class="container"> <div class="starter-template"> <h1>Spring Boot Web Thymeleaf Example</h1> <h2> <span th:text="'Hello, ' + ${message}"></span> </h2> </div> <ol> <li th:each="task : ${tasks}" th:text="${task}"></li> </ol> </main> <!-- /.container --> <script type="text/javascript" th:src="@{webjars/bootstrap/4.2.1/js/bootstrap.min.js}"></script> </body> </html>
5.2 Spring Boot common application properties
welcome.message: Mkyong spring.thymeleaf.cache=false
5.3 For Static files like CSS or JS, put in src/main/resources/static/
body { padding-top: 5rem; .starter-template { padding: 3rem 1.5rem; text-align: center; h1{ color:#0000FF; h2{ color:#FF0000;
6. Unit Test
MockMvc to test the Spring MVC controller.
package com.mkyong; import com.mkyong.controller.WelcomeController; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest; import org.springframework.test.context.junit4.SpringRunner; import org.springframework.test.web.servlet.MockMvc; import org.springframework.test.web.servlet.MvcResult; import org.springframework.test.web.servlet.ResultActions; import org.springframework.web.servlet.ModelAndView; import java.util.Arrays; import java.util.List; import static org.hamcrest.Matchers.containsString; import static org.hamcrest.Matchers.is; import static org.hamcrest.core.IsEqual.equalTo; import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*; @RunWith(SpringRunner.class) @WebMvcTest(controllers = WelcomeController.class) public class WelcomeControllerTest { @Autowired private MockMvc mockMvc; List<String> expectedList = Arrays.asList("a", "b", "c", "d", "e", "f", "g"); @Test public void main() throws Exception { ResultActions resultActions = mockMvc.perform(get("/")) .andExpect(status().isOk()) .andExpect(view().name("welcome")) .andExpect(model().attribute("message", equalTo("Mkyong"))) .andExpect(model().attribute("tasks", is(expectedList))) .andExpect(content().string(containsString("Hello, Mkyong"))); MvcResult mvcResult = resultActions.andReturn(); ModelAndView mv = mvcResult.getModelAndView(); // // Get request with Param @Test public void hello() throws Exception { mockMvc.perform(get("/hello").param("name", "I Love Kotlin!")) .andExpect(status().isOk()) .andExpect(view().name("welcome")) .andExpect(model().attribute("message", equalTo("I Love Kotlin!"))) .andExpect(content().string(containsString("Hello, I Love Kotlin!")));
7. Demo
$ mvn spring-boot:run : Tomcat initialized with port(s): 8080 (http) : Starting service [Tomcat] : Starting Servlet engine: [Apache Tomcat/9.0.14] : Tomcat started on port(s): 8080 (http) with context path '' Started StartWebApplication in 1.858 seconds (JVM running for 2.222)
URL = http://localhost:8080
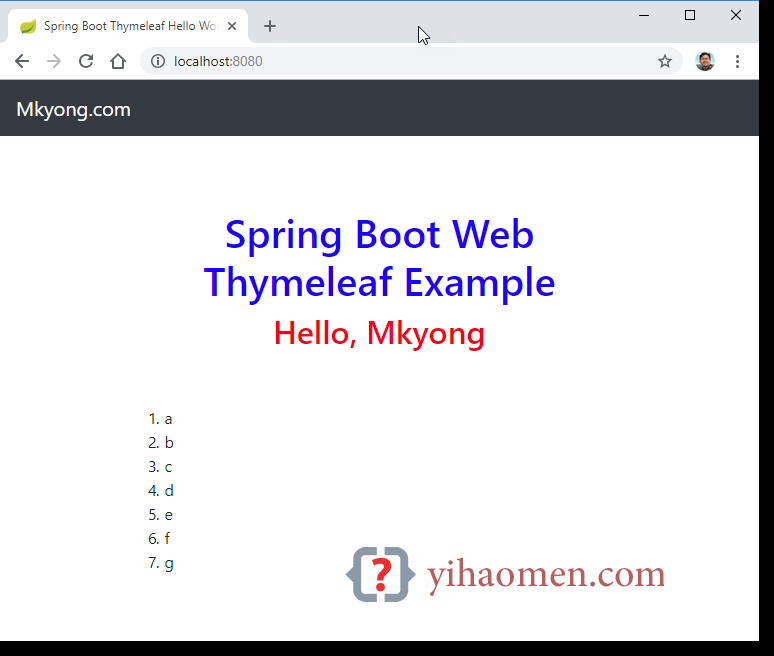
URL = http://localhost:8080/hello?name=abc
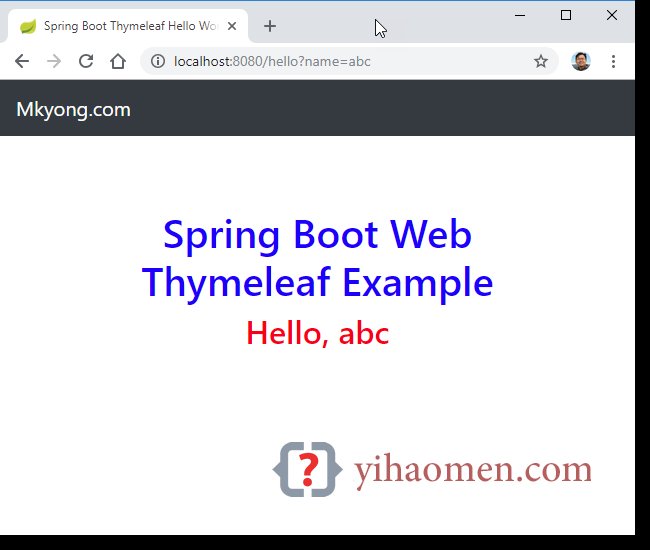
8. Create an executable JAR
For deployment, just normal Maven package to create an executable JAR file.
$ mvn clean package $ java -jar target\web-thymeleaf-1.0.jar
From:一号门
COMMENTS