JSONAssert How to unit test JSON data
By:Roy.LiuLast updated:2019-08-11
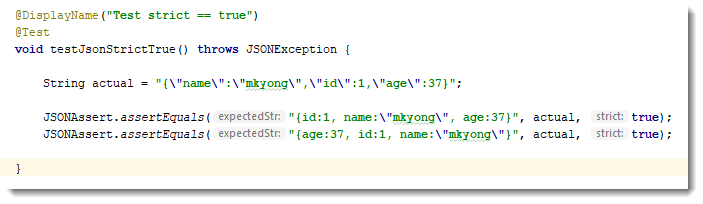
In Java, we can use JSONAssert to unit test JSON data easily.
1. Maven
pom.xml
<dependency> <groupId>org.skyscreamer</groupId> <artifactId>jsonassert</artifactId> <version>1.5.0</version> </dependency>
2. JSON Object
To test the JSON Object, Strict or not, fields order does not matter. If the extended fields are matter, enable the strict mode.
2.1 When the strictMode is off.
JSONObject actual = new JSONObject(); actual.put("id", 1); actual.put("name", "mkyong"); actual.put("age", 37); JSONAssert.assertEquals("{id:1}", actual, false); //Pass, extended fields doesn't matter JSONAssert.assertEquals("{name:\"mkyong\"}", actual, false); //Pass JSONAssert.assertEquals("{name:\"mkyong\", age:37}", actual, false);//Pass JSONAssert.assertEquals("{name:mkyong, id:1}", actual, false); //Pass JSONAssert.assertEquals("{id:1, age:37}", actual, false); //Pass
2.2 When the strictMode is on.
String actual = "{\"name\":\"mkyong\",\"id\":1,\"age\":37}"; JSONAssert.assertEquals("{id:1, name:\"mkyong\", age:37}", actual, true); //Pass, fields order doesn't matter JSONAssert.assertEquals("{age:37, id:1, name:\"mkyong\"}", actual, true); //Pass JSONAssert.assertEquals("{name:\"mkyong\"}", actual, true); // Fail, extended fields must match JSONAssert.assertEquals("{name:\"mkyong\", age:37}", actual, true); // Fail, extended fields must match
3. JSON Array
To test the JSON Array, Strict or not, extended fields must match. If the fields order is matter, enable the strict mode.
3.1 When the strictMode is off.
String result = "[1,2,3,4,5]"; JSONAssert.assertEquals("[1,2,3,4,5]", result, false); // Pass JSONAssert.assertEquals("[5,3,2,1,4]", result, false); // Pass, fields order doesn't matter JSONAssert.assertEquals("[1,2,3,4]", result, false); // Fail, extended fields must match
3.2 When the strictMode is on.
String result = "[1,2,3,4,5]"; JSONAssert.assertEquals("[1,2,3,4,5]", result, true); // Pass JSONAssert.assertEquals("[5,3,2,1,4]", result, true); // Fail, fields order must match. JSONAssert.assertEquals("[1,2,3,4]", result, true); // Fail, extended fields must match
4. Spring Boot + JSONAssert
4.1 For Spring Boot, the JSONAssert is bundled in spring-boot-starter-test
+- org.springframework.boot:spring-boot-starter-test:jar:2.1.2.RELEASE:test [INFO] | +- org.springframework.boot:spring-boot-test:jar:2.1.2.RELEASE:test [INFO] | +- org.springframework.boot:spring-boot-test-autoconfigure:jar:2.1.2.RELEASE:test [INFO] | +- com.jayway.jsonpath:json-path:jar:2.4.0:test [INFO] | | \- net.minidev:json-smart:jar:2.3:test [INFO] | | \- net.minidev:accessors-smart:jar:1.2:test [INFO] | | \- org.ow2.asm:asm:jar:5.0.4:test [INFO] | +- junit:junit:jar:4.12:test [INFO] | +- org.assertj:assertj-core:jar:3.11.1:test [INFO] | +- org.mockito:mockito-core:jar:2.23.4:test [INFO] | | +- net.bytebuddy:byte-buddy-agent:jar:1.9.7:test [INFO] | | \- org.objenesis:objenesis:jar:2.6:test [INFO] | +- org.hamcrest:hamcrest-core:jar:1.3:test [INFO] | +- org.hamcrest:hamcrest-library:jar:1.3:test [INFO] | +- org.skyscreamer:jsonassert:jar:1.5.0:test <<-------- JSONAssert [INFO] | | \- com.vaadin.external.google:android-json:jar:0.0.20131108.vaadin1:test [INFO] | \- org.xmlunit:xmlunit-core:jar:2.6.2:test
4.2 A simple Spring boot integration test with JSONAssert
package com.mkyong; import org.json.JSONException; import org.junit.Test; import org.junit.runner.RunWith; import org.skyscreamer.jsonassert.JSONAssert; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.boot.test.mock.mockito.MockBean; import org.springframework.boot.test.web.client.TestRestTemplate; import org.springframework.http.*; import org.springframework.test.context.ActiveProfiles; import org.springframework.test.context.junit4.SpringRunner; import static org.junit.Assert.assertEquals; import static org.mockito.ArgumentMatchers.any; import static org.mockito.Mockito.times; import static org.mockito.Mockito.verify; @RunWith(SpringRunner.class) @SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT) // for restTemplate @ActiveProfiles("test") public class BookControllerRestTemplateTest { private static final ObjectMapper om = new ObjectMapper(); @Autowired private TestRestTemplate restTemplate; @MockBean private BookRepository mockRepository; /* "timestamp":"2019-03-05T09:34:13.207+0000", "status":400, "errors":["Author is not allowed."] */ @Test public void save_invalidAuthor_400() throws JSONException { String bookInJson = "{\"name\":\" Spring REST tutorials\", \"author\":\"abc\",\"price\":\"9.99\"}"; HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_JSON); HttpEntity<String> entity = new HttpEntity<>(bookInJson, headers); //Try exchange ResponseEntity<String> response = restTemplate.exchange("/books", HttpMethod.POST, entity, String.class); String expectedJson = "{\"status\":400,\"errors\":[\"Author is not allowed.\"]}"; assertEquals(HttpStatus.BAD_REQUEST, response.getStatusCode()); JSONAssert.assertEquals(expectedJson, response.getBody(), false); verify(mockRepository, times(0)).save(any(Book.class));
P.S Tested with Spring Boot 2
From:一号门
Previous:Spring REST Error Handling Example
COMMENTS