Java Generate random integers in a range
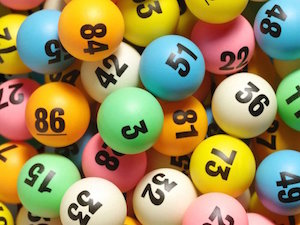
In this article, we will show you three ways to generate random integers in a range.
- java.util.Random.nextInt
- Math.random
- java.util.Random.ints (Java 8)
1. java.util.Random
This Random().nextInt(int bound) generates a random integer from 0 (inclusive) to bound (exclusive).
1.1 Code snippet. For getRandomNumberInRange(5, 10), this will generates a random integer between 5 (inclusive) and 10 (inclusive).
private static int getRandomNumberInRange(int min, int max) { if (min >= max) { throw new IllegalArgumentException("max must be greater than min"); Random r = new Random(); return r.nextInt((max - min) + 1) + min;
1.2 What is (max – min) + 1) + min?
Above formula will generates a random integer in a range between min (inclusive) and max (inclusive).
//Random().nextInt(int bound) = Random integer from 0 (inclusive) to bound (exclusive) //1. nextInt(range) = nextInt(max - min) new Random().nextInt(5); // [0...4] [min = 0, max = 4] new Random().nextInt(6); // [0...5] new Random().nextInt(7); // [0...6] new Random().nextInt(8); // [0...7] new Random().nextInt(9); // [0...8] new Random().nextInt(10); // [0...9] new Random().nextInt(11); // [0...10] //2. To include the last value (max value) = (range + 1) new Random().nextInt(5 + 1) // [0...5] [min = 0, max = 5] new Random().nextInt(6 + 1) // [0...6] new Random().nextInt(7 + 1) // [0...7] new Random().nextInt(8 + 1) // [0...8] new Random().nextInt(9 + 1) // [0...9] new Random().nextInt(10 + 1) // [0...10] new Random().nextInt(11 + 1) // [0...11] //3. To define a start value (min value) in a range, // For example, the range should start from 10 = (range + 1) + min new Random().nextInt(5 + 1) + 10 // [0...5] + 10 = [10...15] new Random().nextInt(6 + 1) + 10 // [0...6] + 10 = [10...16] new Random().nextInt(7 + 1) + 10 // [0...7] + 10 = [10...17] new Random().nextInt(8 + 1) + 10 // [0...8] + 10 = [10...18] new Random().nextInt(9 + 1) + 10 // [0...9] + 10 = [10...19] new Random().nextInt(10 + 1) + 10 // [0...10] + 10 = [10...20] new Random().nextInt(11 + 1) + 10 // [0...11] + 10 = [10...21] // Range = (max - min) // So, the final formula is ((max - min) + 1) + min //4. Test [10...30] // min = 10 , max = 30, range = (max - min) new Random().nextInt((max - min) + 1) + min new Random().nextInt((30 - 10) + 1) + 10 new Random().nextInt((20) + 1) + 10 new Random().nextInt(21) + 10 //[0...20] + 10 = [10...30] //5. Test [15...99] // min = 15 , max = 99, range = (max - min) new Random().nextInt((max - min) + 1) + min new Random().nextInt((99 - 15) + 1) + 15 new Random().nextInt((84) + 1) + 15 new Random().nextInt(85) + 15 //[0...84] + 15 = [15...99] //Done, understand?
1.3 Full examples to generate 10 random integers in a range between 5 (inclusive) and 10 (inclusive).
package com.mkyong.example.test; import java.util.Random; public class TestRandom { public static void main(String[] args) { for (int i = 0; i < 10; i++) { System.out.println(getRandomNumberInRange(5, 10)); private static int getRandomNumberInRange(int min, int max) { if (min >= max) { throw new IllegalArgumentException("max must be greater than min"); Random r = new Random(); return r.nextInt((max - min) + 1) + min;
Output.
10 10
2. Math.random
This Math.random() gives a random double from 0.0 (inclusive) to 1.0 (exclusive).
2.1 Code snippet. Refer to 1.2, more or less it is the same formula.
(int)(Math.random() * ((max - min) + 1)) + min
2.2 Full examples to generate 10 random integers in a range between 16 (inclusive) and 20 (inclusive).
package com.mkyong.example.test; public class TestRandom { public static void main(String[] args) { for (int i = 0; i < 10; i++) { System.out.println(getRandomNumberInRange(16, 20)); private static int getRandomNumberInRange(int min, int max) { if (min >= max) { throw new IllegalArgumentException("max must be greater than min"); return (int)(Math.random() * ((max - min) + 1)) + min;
Output.
17 16 20 19 20 20 20 17 20 16
The Random.nextInt(n) is more efficient than Math.random() * n, read this Oracle forum post.
3. Java 8 Random.ints
In Java 8, new methods are added in java.util.Random
public IntStream ints(int randomNumberOrigin, int randomNumberBound) public IntStream ints(long streamSize, int randomNumberOrigin, int randomNumberBound)
This Random.ints(int origin, int bound) or Random.ints(int min, int max) generates a random integer from origin (inclusive) to bound (exclusive).
3.1 Code snippet.
private static int getRandomNumberInRange(int min, int max) { Random r = new Random(); return r.ints(min, (max + 1)).findFirst().getAsInt();
3.2 Full examples to generate 10 random integers in a range between 33 (inclusive) and 38 (inclusive).
package com.mkyong.form.test; import java.util.Random; public class TestRandom { public static void main(String[] args) { for (int i = 0; i < 10; i++) { System.out.println(getRandomNumberInRange(33, 38)); private static int getRandomNumberInRange(int min, int max) { Random r = new Random(); return r.ints(min, (max + 1)).limit(1).findFirst().getAsInt();
Output.
34 35 37 33 38 37 34 35 36 37
3.3 Extra, for self-reference.
Generates random integers in a range between 33 (inclusive) and 38 (exclusive), with stream size of 10. And print out the items with forEach.
//Java 8 only new Random().ints(10, 33, 38).forEach(System.out::println);
Output.
34 37 37 34 34 35 36 33 37 34
References
- java.util.Random JavaDoc
- java.lang.Math JavaDoc
- Oracle forum : Random Number Generation
- Generating weighed random numbers in JavaScript
From:一号门
Previous:Java 8 forEach examples
COMMENTS